Importing CSV Files into MongoDB: A Comprehensive Guide
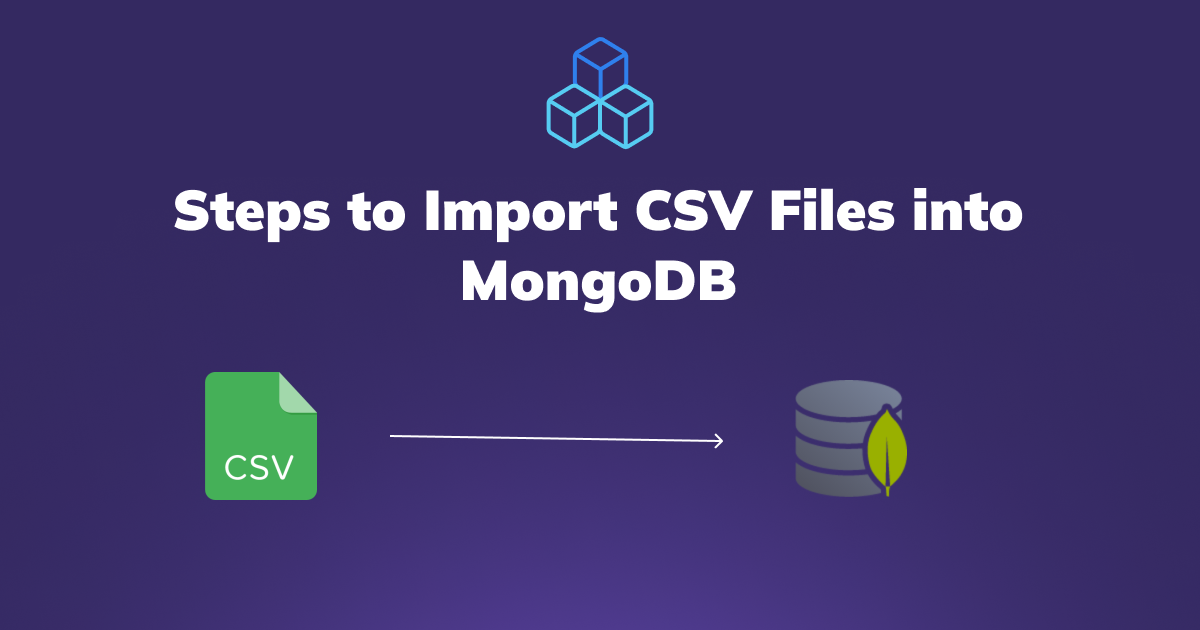
MongoDB, a NoSQL database, is known for its scalability and performance, especially when dealing with large scales of data. Often, some of this data may be stored in CSV (Comma Separated Values) format, which is a widely-used format for data storage and exchange.
In this blog post, we'll explore various ways to import CSV files into MongoDB. This process can be invaluable for data scientists, engineers, and analysts who require an efficient method to import data into a MongoDB database.
Table of Contents
- Introduction to MongoDB and CSV
- Prerequisites
- Using MongoDB's Import Tool (mongoimport)
- Importing CSV with Python's pandas and pymongo
- Using MongoDB's Compass GUI
- Handling Common Issues
- Performance Considerations
- Best Practices
- Conclusion
Introduction to MongoDB and CSV
MongoDB stores data in flexible, JSON-like documents, which makes the amalgamation of diverse data types easier. CSV, on the other hand, is a simple text file that uses a comma to separate values. Each line of the file is a data record, and each record consists of one or more fields separated by commas.
Prerequisites
Before getting started, make sure you have: MongoDB installed and running on your machine. A basic understanding of the terminal or command prompt. Python installed (if using a Python approach).
Using MongoDB's Import Tool (mongoimport)
MongoDB provides a built-in tool called mongoimport for importing data from CSV, TSV (tab-separated values), or JSON files. Here is a step-by-step guide:
- Prepare Your CSV File: Ensure your CSV file has headers that describe the fields.
Example employees.csv:
name,age,department
Alice,30,HR
Bob,24,Engineering
Charlie,28,Sales
- Using mongoimport: Open your terminal or command prompt and execute the following command:
mongoimport --db yourdatabase --collection yourcollection --type csv --headerline --file /path/to/your/employees.csv
Explanation:
--db: Specifies the database.
--collection: Specifies the collection within the database.
--type: Specifies the file type, in this case, csv.
--headerline: Indicates that the first line of the CSV file contains field names.
--file: Path to the CSV file.
This command will import the data into the specified MongoDB collection.
Importing CSV with Python's pandas and pymongo
For those more comfortable with Python, you can use pandas to read the CSV file and pymongo to insert the data into MongoDB. Here is a step-by-step guide:
- Installation: Install the necessary packages if you haven't done so already:
pip install pandas pymongo
- Writing the Python Script: Create a new Python script, for example, import_csv_to_mongodb.py.
import pandas as pd from pymongo
import MongoClient
# Load CSV file into DataFrame
df = pd.read_csv('/path/to/your/employees.csv')
# Connect to MongoDB
client = MongoClient('mongodb://localhost:27017/')
db = client['yourdatabase']
collection = db['yourcollection']
# Convert DataFrame to dictionary and insert into MongoDB
data = df.to_dict(orient='records')collection.insert_many(data)
print("Data imported successfully!")
Explanation:
pandas.read_csv(): Reads the CSV file into a DataFrame.
MongoClient: Connects to the MongoDB server.
[yourdatabase]: Accesses the database.
[yourcollection]: Accesses the collection.
df.to_dict(orient='records'): Converts DataFrame to a dictionary.
insert_many(): Inserts the data into MongoDB.
Run the script: python import_csv_to_mongodb.py
Using MongoDB's Compass GUI
MongoDB Compass is a graphical user interface that makes it easier to interact with MongoDB. You can also use Compass to import CSV files into your database:
- Open MongoDB Compass: Open MongoDB Compass and connect to your database.
- Import Data: Go to the database and collection where you want to import the data. Click on the "Collection" tab. Click on the "Add Data" button and select "Import File". Choose your CSV file. Map the fields if necessary. Click "Import". This method is very user-friendly, especially for those who prefer a GUI over the command-line interface.
Handling Common Issues
Despite how straightforward importing CSV files into MongoDB can be, you might encounter some common issues: Handling Data Types: CSV files store all data as strings. MongoDB, however, supports various data types including integers, dates, and arrays. You might need to convert these data types manually after import.
Example with Python:
# Convert age to integer
df['age'] = df['age'].astype(int)
Performance Considerations
When importing a large CSV file, performance can be an issue. Below are steps that can help you with performance issues:
- Batch Insertions: Instead of inserting records one by one, use batch insertions. This can significantly speed up the import process.
- Indexing: Indexes improve the speed of read operations but can slow down write operations. Consider dropping the indexes before the import and recreating them afterwards.
Best Practices
- Validate Data: Ensure your CSV data is clean and follows the expected schema.
- Backup: Always back up your MongoDB data before performing bulk imports.
- Logging: Enable logging to monitor the import process.
- Error Handling: Implement proper error handling in your scripts or commands.
Conclusion
Importing CSV files into MongoDB can be achieved through various methods, including the built-in mongoimport tool, Python's pandas and pymongo, and the MongoDB Compass GUI. Each method has its pros and cons, and the choice depends on your specific needs and expertise.
By following the steps and best practices outlined in this guide, you will be well-equipped to import CSV data into MongoDB efficiently and effectively.
Whether you're dealing with small datasets or large-scale data migration, mastering the art of importing CSV into MongoDB is an essential skill that can significantly enhance your data handling capabilities.