9 Top CSV Parser Libraries: Efficient Data Processing at Your Fingertips
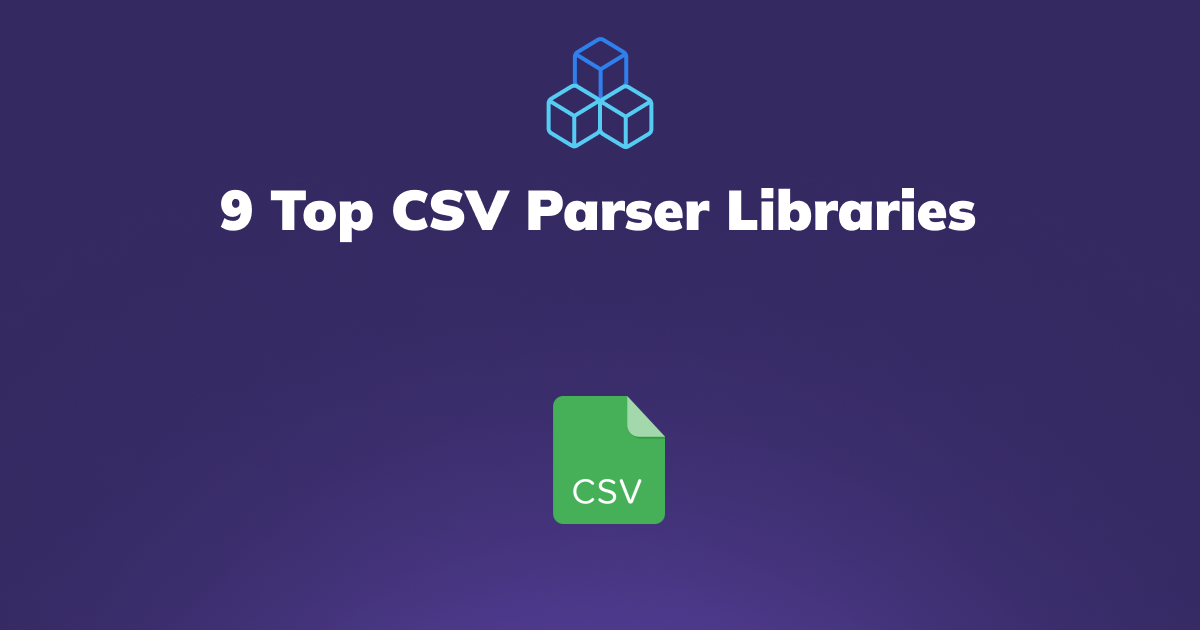
In modern data-driven environments, CSV (Comma-Separated Values) files have become indispensable. Whether you're handling data analytics, machine learning projects, or simply migrating data between platforms, the ability to parse and manipulate CSV files efficiently is crucial.
This article endeavors to guide you through some of the best CSV parser libraries available, each tailored to meet different needs and ease the complexities of CSV parsing.
Understanding CSV and Its Importance
CSV is a simple file format used to store tabular data, such as a database or spreadsheet. Each line of the file is a data record, and each record consists of one or more fields, separated by commas. Due to its simplicity and widespread adoption, CSV has become a ubiquitous format for data exchange and storage. However, the simplicity of CSV also brings challenges.
Despite its straightforward structure, handling special characters, differing line breaks, varying field delimiters, and complex datasets can get tricky. That's where CSV parser libraries come into play. They automate and simplify the task of reading, writing, and manipulating CSV files without getting bogged down by the intricacies of the format.
Criteria for Choosing a CSV Parser Library
When selecting a CSV parser library, there are several key factors to consider:
- Performance: Efficiently handling large datasets.
- Ease of Use: Simplicity and clarity of the API.
- Flexibility: Ability to customize parsing behavior.
- Compatibility: Support for various programming languages and data formats.
- Community and Support: Availability of documentation and community support.
Based on these criteria, let’s explore some of the top CSV parser libraries across different programming languages.
Top CSV Parser Libraries
1. Pandas (Python)
Pandas is a powerhouse when it comes to data analysis and manipulation in Python. While not solely a CSV parser, it's read_csv()
and to_csv()
methods are incredibly efficient, making it a go-to library for handling CSV files.
Key Features
- High Performance: Optimized for large datasets using power-packed data structures.
- Flexibility: Supports complex operations like filtering, grouping, and merging.
- Ease of Use: Intuitive API with detailed documentation.
Example
import pandas as pd
# Reading a CSV file
data_frame = pd.read_csv('data.csv')
# Writing to a CSV file
data_frame.to_csv('output.csv', index=False)
2. csv-parse (Node.js)
Overviewcsv-parse is part of a suite of libraries known as "CSV for Node.js." It is renowned for its performance and adaptability, making it a solid choice for JavaScript developers.
Key Features
- Async Parsing: Supports asynchronous parsing out-of-the-box.
- Custom Delimiters: Easily parse files with different delimiters.
- Event-Driven: Leveraging Node.js's event-driven architecture for efficient data handling.
Example
const parse = require('csv-parse');
const fs = require('fs');
fs.createReadStream('data.csv')
.pipe(parse({ delimiter: ',' }))
.on('data', (row) => {
console.log(row);
})
.on('end', () => {
console.log('CSV file successfully processed');
});
3. OpenCSV (Java)
OpenCSV is a popular library for processing CSV files in Java, providing a simple yet powerful set of functionalities for CSV parsing and writing.
Key Features
- Annotation Support: Use annotations to map CSV columns to Java object fields.
- Robust Parsing: Handles special characters, quotes, and escape characters efficiently.
- Bean Integration: Easily convert CSV data to JavaBeans.
Example
import com.opencsv.CSVReader;
import java.io.FileReader;
import java.io.IOException;
public class CSVParserExample {
public static void main(String[] args) {
try (CSVReader reader = new CSVReader(new FileReader("data.csv"))) {
String[] line;
while ((line = reader.readNext()) != null) {
System.out.println("Column 1: " + line[0] + ", Column 2: " + line[1]);
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
4. CSVHelper (C#)
CSVHelper is a versatile library for reading and writing CSV files in C#. It stands out for its ease of use and ability to handle complex CSV structures seamlessly.
Key Features
- Automatic Mapping: Automatically maps CSV columns to class properties.
- Customizable: High customization options for delimiter, header, and formatting.
- Culture-Sensitive: Supports different cultures and custom type conversions.
Example
using CsvHelper;using System.Globalization;using System.IO;
public class CSVParserExample{
public void ParseCSV()
{
using (var reader = new StreamReader("data.csv"))
using (var csv = new CsvReader(reader, CultureInfo.InvariantCulture))
{
var records = csv.GetRecords<Record>().ToList();
foreach (var record in records)
{
Console.WriteLine($"{record.Column1}, {record.Column2}");
}
}
}
}
public class Record{
public string Column1 { get; set; }
public string Column2 { get; set; }
}
5. FastCSV (JavaScript)
FastCSV is a lightweight JavaScript library optimized for speed and minimal memory usage. It's perfect for in-browser CSV parsing.
Key Features
- Small Footprint: Minimal impact on page load times.
- Speed: Optimized for fast parsing.
- Browser Compatibility: Works seamlessly in modern web browsers.
Example
import { parse } from '@fast-csv/parse';
import { createReadStream } from 'fs';
createReadStream('data.csv').pipe(parse({ headers: true }))
.on('data', row => { console.log(row); })
.on('end', () => { console.log('CSV file successfully processed'); });
6. Ruby's CSV Library
Ruby’s standard library includes a CSV module that provides comprehensive tools for reading and writing CSV files.
Key Features
- Simplicity: Easy to use with Ruby’s elegant syntax.
- Flexibility: Handles different delimiters, quotes, and encodings.
- Built-in: Included in Ruby’s standard library.
Example
require 'csv'
CSV.foreach('data.csv', headers: true) do
|row| puts row['Column1']
end
7. Apache Commons CSV (Java)
Apache Commons CSV is part of the Apache Commons project. It’s a powerful and flexible library for processing CSV files in Java.
Key Features
- Robustness: Handles malformed data and different quote characters.
- Customizable: Supports various formatting options.
- Part of Apache Commons: Benefits from the reliability and extensive documentation of Apache Commons libraries.
Example
import org.apache.commons.csv.CSVFormat;import org.apache.commons.csv.CSVParser;import org.apache.commons.csv.CSVRecord;
import java.io.FileReader;import java.io.IOException;
public class CSVParserExample {
public static void main(String[] args) {
try (FileReader reader = new FileReader("data.csv");
CSVParser csvParser = new CSVParser(reader, CSVFormat.DEFAULT.withHeader())) {
for (CSVRecord record : csvParser) {
System.out.println(record.get("Column1") + ", " + record.get("Column2"));
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
8. Tablib (Python)
Tablib is another powerful library for Python that handles tabular data. Although not limited to CSV, it offers strong support for reading and writing CSV files.
Key Features
- Multi-format Support: Handles CSV, JSON, XLSX, and more.
- Simplicity: Easy to use with intuitive API.
- Cross-Compatible: Works well with Django, Flask, and other frameworks.
Example
import tablib
data = tablib.Dataset()
data.csv = open('data.csv').read()
print(data.dict)
9. CSV. (JavaScript)
CSV is a suite of libraries in JavaScript optimized for CSV manipulation. It combines performance with an easy-to-use API.
Key Features
- Suite of Libraries: Comprehensive set of CSV tools.Optimized
- Performance: Efficient parsing and writing.
- Modularized: Use only what you need for your application.
Example
const { parse } = require('csv');
const fs = require('fs');
fs.createReadStream('data.csv')
.pipe(parse())
.on('data', (row) => { console.log(row); })
.on('end', () => { console.log('CSV file successfully processed'); });
Conclusion
Selecting the right CSV parser library can significantly streamline your data processing tasks, making it crucial to choose one that aligns with your performance requirements, ease of use, and flexibility needs.
Whether you’re working in Python, JavaScript, Java, or C#, there are robust options available to meet your needs.
Final Thoughts:
The landscape of CSV parser libraries is vast and varied. The key to efficient data manipulation lies in understanding the specific requirements of your project and leveraging the strengths of the libraries discussed above.
Whether you're working on complex data analysis with Pandas, building a Node.js application with csv-parse, or handling data in Java with OpenCSV, the right choice of library can save you time and effort, turning raw data into actionable insights seamlessly.
As the world continues to generate vast amounts of data, the role of efficient data processing tools becomes ever more essential.
With the right CSV parser library in your toolkit, you'll be well-equipped to manage, analyze, and utilize data effectively, driving better decisions and innovation in your projects.